- Skip to main content
- Select language
- Skip to search

ReferenceError: assignment to undeclared variable "x"
Valid cases.
ReferenceError warning in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
To make "bar" a declared variable, you can add the var keyword in front of it.
- Strict mode
Document Tags and Contributors
- ReferenceError
- Strict Mode
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting a property that has only a getter
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

Next-Gen App & Browser Testing Cloud
Trusted by 2 Mn+ QAs & Devs to accelerate their release cycles
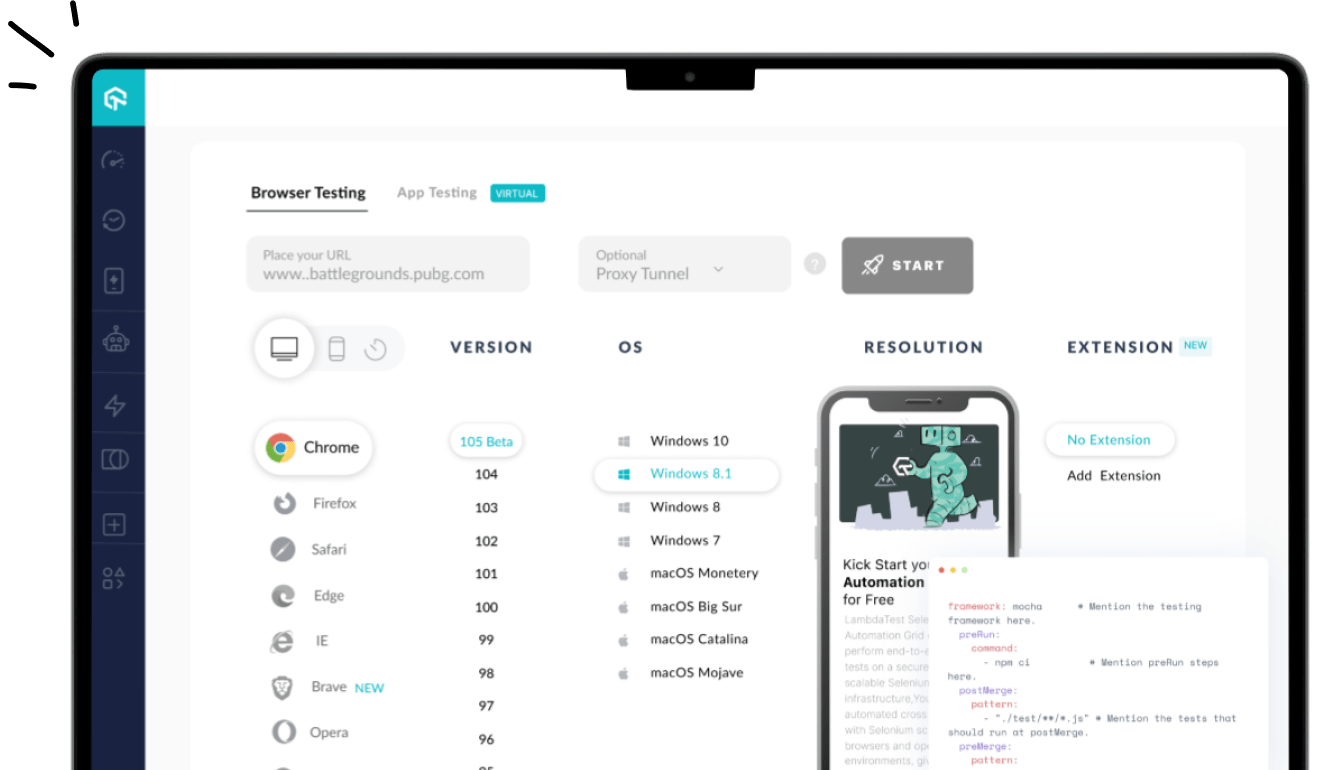
Debug JavaScript Miscellaneous
- Reference Error: JavaScript
Posted On: April 17, 2018

How does it feel when you go to give a job interview and after reaching the interview location you find out that the company for which you are here doesn’t even exist.
Obviously you got angry and your mind will start throwing negative thoughts.
Exactly same happens with JavaScript too.
When any value is assigned to undeclared variable or assignment without the var keyword or variable is not in your current scope, it might lead to unexpected results and that’s why JavaScript presents a ReferenceError: assignment to undeclared variable "x" in strict mode. And this error causes a problem in execution of functions.
If you’ve begun to try out JavaScript you might have encountered some pretty baffling errors. I know I sure did…
ReferenceError: assignment to undeclared variable “x”
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Code without ‘var’ keyword
foo() { 'use strict'; bar = true; //variable not declared (); |
What you get after executing above program?? An Error?? 🙁

How do you need to code 🙂
Insert ‘var’ in front of your variable and see your program running
foo() { 'use strict'; var bar = true; //declared variable here (); |
Likewise there are many scripting factors possible to generate reference error in javascript.
: "x" is not defined |
: deprecated caller or arguments usage |
: can't access lexical declaration`X' before initialization |
: reference to undefined property "x" |
: invalid assignment left-hand side |
Saif Sadiq is a Product Growth specialist at LambdaTest and Growth Marketer.
See author's profile

Author’s Profile

Got Questions? Drop them on LambdaTest Community. Visit now
Test Your Web Or Mobile Apps On 3000+ Browsers
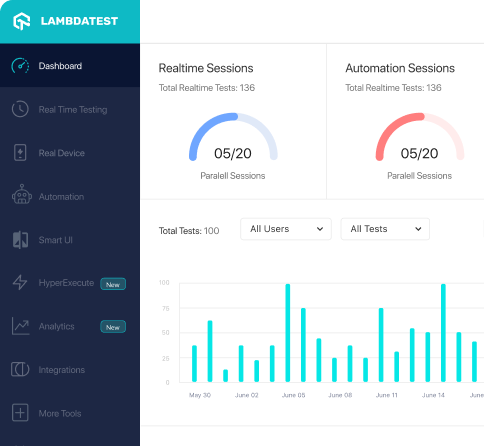
Related Articles

How to Unblock a Website: Step-by-Step Guide
Salman Khan
June 20, 2024
Miscellaneous |
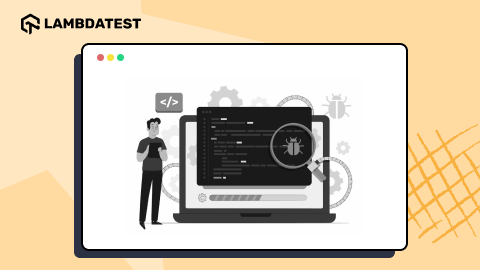
How To Find Bugs In Website?
Hari Sapna Nair
May 2, 2024
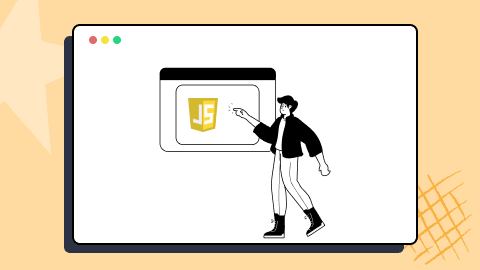
27 Best JavaScript Frameworks For 2024
Mehul Gadhiya
April 29, 2024
JavaScript | Web Development |
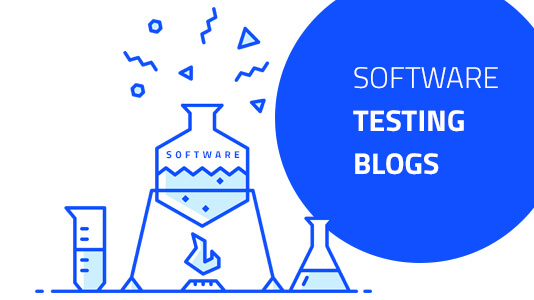
Top 17 Software Testing Blogs [2024]
Arnab Roy Chowdhury
March 27, 2024
Miscellaneous | Recent Blogs |
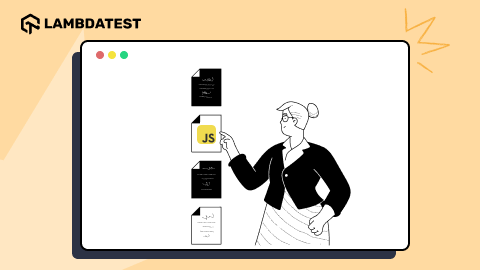
Choosing The Right JavaScript Frameworks In 2024
Robin Jangu
March 22, 2024
JavaScript | Miscellaneous |
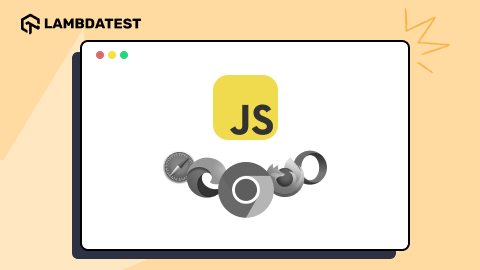
How to Solve Cross Browser Compatibility Issues in JavaScript
Suraj Kumar
March 21, 2024
Cross Browser Testing | JavaScript |
Try LambdaTest Now !!
Get 100 minutes of automation test minutes FREE!!
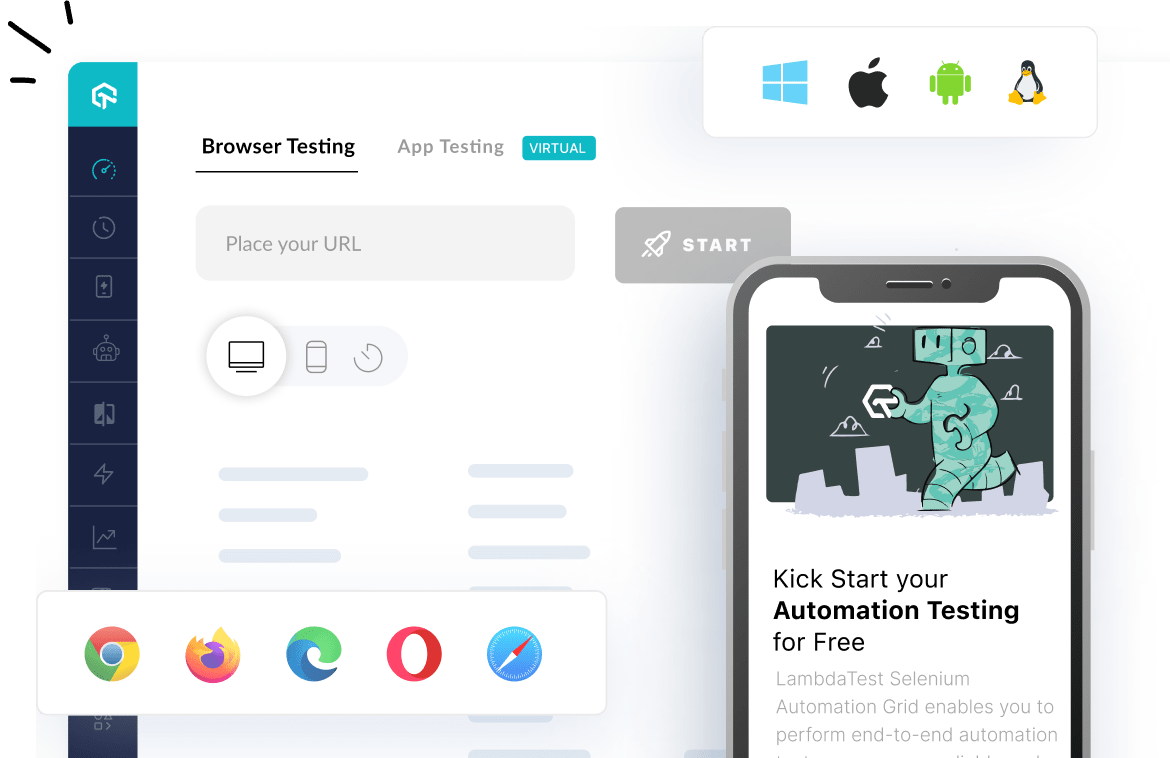
Download Whitepaper
You'll get your download link by email.
Don't worry, we don't spam!
We use cookies to give you the best experience. Cookies help to provide a more personalized experience and relevant advertising for you, and web analytics for us. Learn More in our Cookies policy , Privacy & Terms of service .
Schedule Your Personal Demo ×
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
ReferenceError: assignment to undeclared variable "x"
The JavaScript strict mode -only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add a let , const , or var keyword in front of it.
- Strict mode
ReferenceError: assignment to undeclared variable "x"
The JavaScript strict mode -only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add a let , const , or var keyword in front of it.
- Strict mode
© 2005–2023 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Errors/Undeclared_var
- Skip to main content
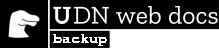
ReferenceError: assignment to undeclared variable "x"
The JavaScript strict mode -only exception "Assignment to undeclated variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError warning in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add the var keyword in front of it.
- Strict mode
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side JavaScript frameworks
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- AggregateError
- ArrayBuffer
- AsyncFunction
- BigInt64Array
- BigUint64Array
- FinalizationRegistry
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) operator
- Destructuring assignment
- Function expression
- Grouping operator
- Logical AND
- Logical NOT
- Logical operators
- Nullish coalescing operator
- Object initializer
- Operator precedence
- Optional chaining
- Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function* expression
- in operator
- new operator
- void operator
- async function
- for await...of
- function declaration
- import.meta
- try...catch
- Arrow function expressions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- Private class fields
- Public class fields
- constructor
- Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration "x" before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the "delete" operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: "x" is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: X.prototype.y called on incompatible type
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use "in" operator to search for "x" in "y"
- TypeError: cyclic object value
- TypeError: invalid "instanceof" operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features

- Get Started
Jan 3, 2017 11:34:16 AM | JavaScript Error Handling - ReferenceError: "x" is not defined
The Undefined Variable error, occurs when variables or object are referenced in code that doesn't exist, or is out of scope of the executing code.
Next up on the list of articles in our JavaScript Error Handling series we take a closer look at the Undefined Variable error. The Undefined Variable error is thrown when a reference to a variable or object is made in code that either doesn't exist, or is outside the scope of the executing code.
Below we'll take a look at a couple of specific examples that will commonly produce a Undefined Variable error, as well as how to catch and deal with this error when it appears. Let's get started!
The Technical Rundown
- All JavaScript error objects are descendants of the Error object, or an inherited object therein.
- The ReferenceError object is inherited from the Error object.
- The Undefined Variable error is a specific type of ReferenceError object.
When Should You Use It?
When deep in the process of coding with JavaScript, it isn't all that unheard of to make a typo or simply forget to initialize a variable or object before calling said variable later down the line. When this occurs, JavaScript will show its displeasure by throwing a Undefined Variable error, indicating that the referenced object was not previously defined.
For example, here we're making a simple statement of attempting to grab the .length property of our undefined itemvariable. We're also using a simple try-catch block and grabbing any ReferenceErrors that might occur, then passing them along to a simple printError function to beautify the output of our error messages:
try { // Calling an undefined `item `variable var length = item.length; console.log(`Length is ${length}.`) } catch (e) { if (e instanceof ReferenceError) { printError(e, true); } else { printError(e, false); } }
Sure enough, as expected, JavaScript notices that the item variable is undefined, and produces the explicit Undefined Variable error:
// CHROME [EXPLICIT] ReferenceError: item is not defined
It's worth noting that unlike many other JavaScript errors we've covered in this series, the Undefined Variable error message text does not differ between the two engines powering Firefox or Chrome.
The obvious and simple fix to this particular Undefined Variable error is to simply declare our item variable prior to calling it:
try { // Defining `item` first var item = "Bob"; var length = item.length; console.log(`Length is ${length}.`) } catch (e) { if (e instanceof ReferenceError) { printError(e, true); } else { printError(e, false); } }
Now we get past the item.length call without throwing any errors and thus produce our console.log output of the length of our item string:
Technically, while the Undefined Variable error is intended to identify references to undefined variables, it also plays a role when attempting to reference variables that are defined, but are outside of the current scope context where the code is being executed.
For example, here we have a simple getFullName function, which defines two variables inside itself, firstName and lastName. Outside of that function's scope, we attempt to get the length property of the firstName variable:
var printError = function(error, explicit) { console.log(`[${explicit ? 'EXPLICIT' : 'INEXPLICIT'}] ${error.name}: ${error.message}`); }
try { // Accessing `firstName` from outside its scope var length = firstName.length; console.log(`Length is ${length}.`) } catch (e) { if (e instanceof ReferenceError) { printError(e, true); } else { printError(e, false); } }
While the firstName variable is technically defined already, it is inaccessible to us at this level of execution, and thus a Undefined Variable error is thrown:
In this case, resolution is a matter of pulling the firstName and lastName variable outside the scope of the getFullNamefunction, so they are within the same context of execution as our try-catch block:
try { // Accessing `firstName` is now allowed var length = firstName.length; console.log(`Length is ${length}.`) } catch (e) { if (e instanceof ReferenceError) { printError(e, true); } else { printError(e, false); } }
As expected, no errors are produced and we get the length of the firstName variable as output:
To dive even deeper into understanding how your applications deal with JavaScript Errors, check out the revolutionary Airbrake JavaScript error tracking tool for real-time alerts and instantaneous insight into what went wrong with your JavaScript code.
Written By: Frances Banks
You may also like.
Dec 28, 2016 8:00:56 AM | JavaScript Error Handling - ReferenceError: assignment to undeclared variable “x”
May 13, 2017 8:00:02 am | javascript error handling - syntaxerror: redeclaration of formal parameter "x", jun 25, 2017 9:00:31 am | javascript error handling - variable x redeclares argument typeerror.
© Airbrake. All rights reserved. Terms of Service | Privacy Policy | DPA
ReferenceError: assignment to undeclared variable "x"
ReferenceError warning in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.

Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add the var keyword in front of it.
- Strict mode
© 2016 Mozilla Contributors Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-us/docs/web/javascript/reference/errors/undeclared_var
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
What are undeclared and undefined variables in JavaScript?
Undefined: It occurs when a variable has been declared but has not been assigned any value. Undefined is not a keyword.
Undeclared: It occurs when we try to access any variable that is not initialized or declared earlier using the var or const keyword . If we use ‘typeof’ operator to get the value of an undeclared variable, we will face the runtime error with the return value as “undefined” . The scope of the undeclared variables is always global.
For example:
Undefined:
Undeclared:
Example 1: This example illustrates a situation where an undeclared variable is used.
Output:
Example 2: This example checks whether a given variable is undefined or not.
Please Login to comment...
Similar reads.
- Web Technologies
- JavaScript-Questions
- Best Twitch Extensions for 2024: Top Tools for Viewers and Streamers
- Discord Emojis List 2024: Copy and Paste
- Best Adblockers for Twitch TV: Enjoy Ad-Free Streaming in 2024
- PS4 vs. PS5: Which PlayStation Should You Buy in 2024?
- Full Stack Developer Roadmap [2024 Updated]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
热门关键词: Web前端 | 前端开发 | HTML5 | CSS3 | jQuery | CSS3动画
- clip-path生成器
分析出现ReferenceError: assignment to undeclared variable "x"的原因
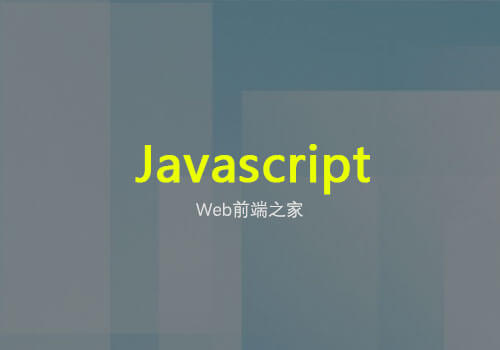
分析出现ReferenceError: assignment to undeclared variable "x"的原因。
我们在开发项目的时候,出现以下报错信息:
仅在严格模式中出现 ReferenceError 警告。
在代码里赋值了一个未声明的变量。换句话说,有处没有带着 var 关键字的赋值。事实上已声明的和未声明的变量之间有一些差异,这可能会导致意想不到的结果,这就是为什么 JavaScript 在严格模式打印出这种错误。
关于已声明和未声明的变量,其有三个注意事项:
已声明的变量被约束在其执行上下文中。未声明的变量总是全局的。
已声明的变量在执行任何代码之前就创建了。未声明的变量则不存在,直到执行相关的代码。
已声明的变量是其执行上下文(函数或全局)的不可配置属性。而未声明的变量是可配置的(例如可以删除)。
更多信息及例子,请参考 var 页面。
关于未声明变量的赋值的错误仅在严格模式里出现。在非严格模式中,这些将被忽略。
在本例中,"bar" 是未声明的变量。
为了使 "bar" 是一个已声明变量,你需要在其前面加一个 var 关键字。
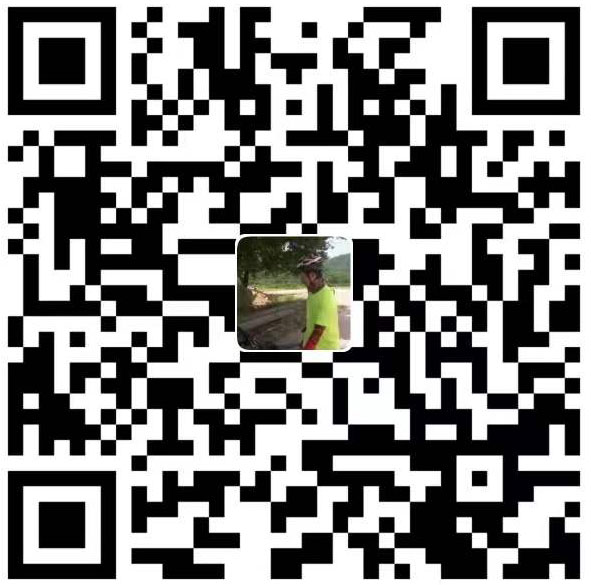
※大家的支持是我们创作的动力!
网友评论 文明上网理性发言 已有0人参与
- to navigate
Search by FlexSearch
Example Reference
Referenceerror: assignment to undeclared variable "x".
The JavaScript strict mode -only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add a let , const , or var keyword in front of it.
- Strict mode
© 2005–2023 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Errors/Undeclared_var
Get the Reddit app
The subreddit for all things related to Modded Minecraft for Minecraft Java Edition --- This subreddit was originally created for discussion around the FTB launcher and its modpacks but has since grown to encompass all aspects of modding the Java edition of Minecraft. The /r/feedthebeast subreddit is not affiliated or associated with the Feed the Beast company.
KubeJS script to unify items in various tags (oreDict) for the latest versions of Minecraft
Everyone knows how annoying it was to have four different copper, tin, silver, etc. ingots from various different mods, and how oreDict helped to mitigate that problem.
Still, it was inconvenient to hold around three or four different types of the same metal that should obviously stack; UniDict and InstantUnify did a good job at solving that issue as well. Today, however, neither of these mods are up-to-date.
So I made a KubeJS script that basically does what InstantUnify does with a little bit of UniDict mixed in, which I call UnifyTags . It will change any items in a tag that are in your inventory or in entity form instantly into the first item in the tag (or based on custom mod priorities). It also modifies crafting, smelting, and [insert KubeJS recipe supported mod here] recipes to accept any item in the tag and spit out the proper item in the tag.
It's all server-side, except for an optional client side script that can hide items in JEI. I'm not sure if the client-side script works if you play on a server first (as it relies upon variables defined in the server-side script in addition to server-side tags), so do be cautious of that. It will show the error, "Failure to hide unified items in JEI. Press F3+T to reload client and retry" in the console if the client-side script fails.
My favorite thing about the script is that it should continue to work regardless of the Minecraft version, provided KubeJS is updated to the latest version. I haven't tested it on anything less than 1.16, but feel free to try!
One final thing to note, if you find any issues, let me know! I want people to be able to keep experiencing the joys of having only a single metal at a time rather than three or four.
UnifyTags - CurseForge - Github
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
The variable 'variable_name' is either undeclared or was never assigned
I have a question related to the error on the title. Im working with c# and Visual Studio 2010.
I have a form declared as "public class FormularioGeneral : Form", which is the base for the rest of the forms in my application. When i try to access the Designer View i get this error several times, as you can see in the image:

But all the variables are declared in the same class as read-only properties and all of them are assigned inside a method which is called in the constructor.
Declaration of properties:
Constructor code:
ConfigurarUI method:
So, as far as i know, all the variable which are giving the errors are correctly declared and have a value assigned before the InitilizeComponent function is called.
Im stuck at this point and dont know what to do to solve the problem. Hope some of you can help me with this issue.
- visual-studio-2010
- windows-forms-designer
- be sure you call your constructor before using your code. public Form1() { FormularioGeneral(); } – Moonlight Commented Dec 15, 2011 at 14:30
- Hello Bruno. The error is produced inside the InitializeComponent method, when i assign the m_* value to some property (for example, when i set the text color of a button --> this.Button1.ForeColor = m_TextButtonColor) – Kitinz Commented Dec 16, 2011 at 10:16
- also discussed here: stackoverflow.com/questions/1915183/… – Christian Commented May 14, 2014 at 13:02
- And here also: stackoverflow.com/questions/12342760/… – Pedro77 Commented May 20, 2015 at 14:27
- 1 I can't write an answer due to not being sure what's going on, and am trying to solve an probably different maybe unrelated problem, but experimenting with my main Solution and an small hackjob custom control project, I find that I must chose "Any CPU" not x86 or x64 to avoid getting these 'variable undeclared' errors. – DarenW Commented Nov 28, 2018 at 22:19
16 Answers 16
So, I have had the same problem in the past, for fix I did the following:
- Solution → Clean Solution;
- Build → Rebuild Solution;
- Close Visual Studio, re-open.
Thanks a lot to Marshall Belew!
- 1 I ways do that, and it works, but I wonder why this error is happening and how can I avoid that. Or this is simple a VS bug? – Pedro77 Commented May 20, 2015 at 14:27
- 4 In case someone finds their way here, the problem might arise from the constructor of the offending control being declared anything other than public . – user1908746 Commented Dec 5, 2016 at 5:14
In my case, I had an older Windows Forms project where InitializeComponents() started like this:
This resulted in an error message later on when accessing the componentResourceManager inside InitializeComponent() :
The variable 'componentResourceManager' is either undeclared or was never assigned.
When comparing with a newly created form, I saw that it was similar to my non-working form, except for one thing:
The variable was not named componentResourceManager but simply resources .
After doing a rename on my variable to also have the name resources , everything works successfully:
The Windows Forms Designer in Visual Studio 2017 did open the form correctly.
- 2 Thanks, worked for me. Can you give any explanation why it worked this way? – Sam Commented Oct 28, 2018 at 10:35
- 1 Amazing! This also worked for me. Can someone please explain why changing to resources works? – tkha007 Commented Oct 8, 2019 at 4:10
- 1 Damn, where did you get that knowledge? Worked like a charm. – Bartosz Commented Jul 12 at 10:17
I ran into this error because my project is x64 only. Apparently Visual Studio, being a 32bit application, cannot load any forms or controls compiled to 64bit in the designer. It makes total sense, but the error gives you no indication that is the problem.
See the answer to Visual studio designer in x64 doesn't work .
The workaround is to change your project to Any CPU when designing, then back when building.
- 2 Too bad even in visual studio 2017 they still don't tell the real reason... 2 hours on the web, after trying manually change the GUI... all that until I came to here... – ephraim Commented Mar 6, 2018 at 8:51
Maybe the error occurs due to your constructor code. Place InitializeComponent(); at the beginning of the constructor like this:
Explanation:
The variables are initialized in that method.
- I already tried it, but this doesnt solve the problem. Anyway, the variables are declared without value. A value is assigned inside the "ConfigurarUI" method (So this method should be executed before using the variable). Finally, in the InitializeComponent the variables are used. – Kitinz Commented Dec 15, 2011 at 14:50
- As far as I understand you correctly, have you changed the code in the InitializeComponent to use your variables? – Fischermaen Commented Dec 15, 2011 at 14:53
- I dont think the assignments were "hardcoded" in the InitializeComponent since it is auto-generated each time you compile. I assume that the values were assigned through the Designer, but cant assure it since other guy started this project and im the "sucessor" :P – Kitinz Commented Dec 15, 2011 at 15:19
- Poor "sucessor". The designer doesn't generate member variables with an "m_" at the beginning. So this was "handmade" and it will make trouble. There is a comment to the "InitializeComponents" method, stating that one should never edit this. Try to solve problem in a different way, maybe by setting the values after InitializeComponents. – Fischermaen Commented Dec 15, 2011 at 15:22
- I know the m_* variables are created manually, but i think you are right. The errors point the assingments made inside the InitializeComponent like "this.Button1.ForeColor = m_TextButtonColor", which should be made through the designer and seems like it was hardcoded. Im going to try to change those hardcoded assingments to this one: "this.Button1.ForeColor = Color.Black" which is the value stored in the m_TextButtonColor :P – Kitinz Commented Dec 16, 2011 at 11:46
I had the same problem and cleaning and rebuilding did not work for me.
In my case the problem was caused by the Visual Studio designer loading referenced DLLs from the GAC instead of loading them from the <HintPath> directory specified in the .csproj file. The DLLs in the GAC did not have the same version as the locally stored DLLs.
When I updated the DLLs in the GAC to have the same version everything worked OK again.
Don't put anything other than InitializeComponent(); in the constructor. You can put the code from there in events like Load() .
I had this issue when my user control had some code in the constructor which was related to runtime resource. I added null check and it fixed.
In my case, I added a third party control in my Toolbar(via a .dll file), and draw one of it in my form. And for some reason, my Toolbar clean this third party control out of the general group(I added it in the general group), so VS cannot find this control. Here is what I done to slove this problem:
- Add this control into the Toolbar.
- Clean the solution
- Rebuild the solution
Redraw the control if neccessary.
User Controls were caused problem and after trying all suggestions, ( Focus solution then Alt+Enter ) changing solution's Platform Target from x64 to Any CPU solved the problem.
About the variables, can you simply initialize them in the declaration? I think that would suffice, even if you change the value later. From what I'm seeing, the compiler is unable to check whether you have initialized them or not because it's not directly on the constructor code, it's being done on a virtual method which will evaluate only at runtime.
So, instead of:
And a comment: you should avoid virtual calls in the constructor, which can lead to obscure errors in your application. Check it here .
- Thanks for the answer. I tried to do as you say, initializing the variables in the declaration, but doesnt solve the problem :P I also read about the virtual methods, and copied all the code in the virtual method inside the constructor, to not need to make the call, but even so, the problem persist :P – Kitinz Commented Dec 16, 2011 at 10:21
This error occurs for me while creating a third party control in InitializeComponent() which is called from form constructor. When I created it after InitializeComponent() it works fine for me.

- This is exactly my problem, I have created some UserControl, and for some reason, the process to generate Design of the Form always complains. After I moved anything seems suspicious out of InitializeComponent, it works fine. Thanks – Tuan Do Commented Jun 25, 2016 at 4:34
I am working with WPF inside of Windows Forms.
I hosted my WPF User Control in a Windows Forms Element Host. Due to that when InitializeComponent() got called I executed Code before reaching the InitializeComponent() of my WPF control. Tricky.
So I moved it out of my constructor, Clean Build, Rebuild, Restart VS and everything works as expected. Finally.
I have had the same problem and I fixed it. Actually Visual Studio only works with X86 controls and you can't create a user control in X64 mode and use it.
You should add a new class library in Any CPU mode and build the class library. then you can add its DLL in your project. Done.
If it doesn't you must go to the Configuration manager and set the Active solution platform to X64 also do that for all subprojects. Remember that build option has to be checked. and go to the properties of the class library and click on the build tab. then set the platform target to Any CPU.

First I had code that referenced something a type that the designer couldn't load (for whatever reason). Then I had code in the constructor that couldn't be executed from my local laptop. I decided the best option was to move the logic to the Load event and check if the component was in DesignMode and exit if it was.
Even this wasn't enough for me as the designer still tried to JIT the type that was later down in the method, so I had to move it out to a separate method to stop that from happening. Here's basically what I ended up with:
Special thanks to this SO answer which pointed me to a comment in a blog post about not accessing the DesignMode property until you're in the Load event...
Renaming the variable componentResourceManager to resources solved error.
Unfortunately i had to change a ton of other items to get the designer working for Telerik reports designer
In my Solution i had wrong Reference paths which i fixed in the .csproj files. After fixing that i could finally load the Form again.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# .net winforms visual-studio-2010 windows-forms-designer or ask your own question .
- The Overflow Blog
- The creator of Jenkins discusses CI/CD and balancing business with open source
- The evolution of full stack engineers
- Featured on Meta
- Bringing clarity to status tag usage on meta sites
- Join Stack Overflow’s CEO and me for the first Stack IRL Community Event in...
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- What is the Kronecker product of two 1D vectors?
- Bathroom fan venting options
- Are there carbon fiber wing spar pins?
- How are you supposed to trust SSO popups in desktop and mobile applications?
- Advice how to prevent sin
- What is the least number of colours Peter could use to color the 3x3 square?
- Circuit that turns two LEDs off/on depending on switch
- Correct anonymization of submission using Latex
- How much does a ma'ah cost in £/$ in today's world?
- How long should a wooden construct burn (and continue to take damage) until it burns out (and stops doing damage)
- When a mass crosses a black hole event horizon does the horizon radius get larger closer to the mass or does it increase equally everywhere?
- Turn on double spacing, but not before equations
- Why does my LED bulb light up dimly when I touch it?
- How to raise and lower indices as a physicist would handle it?
- How to go from Asia to America by ferry
- Do US universities invite faculty applicants from outside the US for an interview?
- What do these expressions mean in NASA's Steve Stitch's brief Starliner undocking statement?
- How to create rounded arrows to highlight inflection points in a TikZ graph?
- Is there a Christian denomination that teaches that God cannot heal?
- Is the white man at the other side of the Joliba river a historically identifiable person?
- Coloring a 4×4 Grid
- Electromagnetic Eigenvalue problem in FEM yielding spurious solutions
- "With" as a function word to specify an additional circumstance or condition
- How to clean a female disconnect spade connector

IMAGES
VIDEO
COMMENTS
ReferenceError: assignment to undeclared variable "x"
ReferenceError: Variable undefined in strict mode (Edge) Invalid cases In this case, the variable "bar" is an undeclared variable. function foo() { 'use strict'; bar = true; } foo(); // ReferenceError: assignment to undeclared variable bar Valid cases To make "bar" a declared variable, you can add the var keyword in front of it.
Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted). For more details and examples, see the var reference page. Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Undefined: It occurs when a variable has been declared but has not been assigned any value. Undefined is not a keyword. Undeclared: It occurs when we try to access any variable that is not initialized or declared earlier using the var or const keyword. If we use 'typeof' operator to get the value of an undeclared variable, we will face the runtime
The Assignment to Undeclared Variable error crops up anytime code attempts to assign a value to a variable that has yet to be declared.
When any value is assigned to undeclared variable or assignment without the var keyword or variable is not in your current scope, it might lead to unexpected results and that's why JavaScript presents a ReferenceError: assignment to undeclared variable "x" in strict mode.
The JavaScript strict mode-only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError: "x" is not defined - JavaScript - MDN Web Docs
Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted). For more details and examples, see the var reference page. Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted). For more details and examples, see the var reference page. Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Sure enough, as expected, JavaScript notices that the item variable is undefined, and produces the explicit Undefined Variable error: // FIREFOX [EXPLICIT] ReferenceError: item is not defined
Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted). For more details and examples, see the var reference page. Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
What are undeclared and undefined variables in JavaScript?
分析出现ReferenceError: assignment to undeclared variable "x"的原因。. 我们在开发项目的时候,出现以下报错信息:. ReferenceError: assignment to undeclared variable "x" (Firefox) ReferenceError: "x" is not defined (Chrome) ReferenceError: Variable undefined in strict mode (Edge)
Undeclared variables are always global. Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed. Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
I'm not sure if the client-side script works if you play on a server first (as it relies upon variables defined in the server-side script in addition to server-side tags), so do be cautious of that. It will show the error, "Failure to hide unified items in JEI. Press F3+T to reload client and retry" in the console if the client-side script fails.
发生了什么?. 在代码里赋值了一个未声明的变量。. 换句话说,有处没有带着 var 关键字的赋值。. 事实上已声明的和未声明的变量之间有一些差异,这可能会导致意想不到的结果,这就是为什么 JavaScript 在严格模式打印出这种错误。. 关于已声明和未声明的变量 ...
For declared global variables, the configurable attribute is false. For undeclared global variables, it's true. The value of the configurable attribute can be retrieved using the getOwnPropertyDescriptor method, as shown below. var declared = 1; undeclared = 1; (Object.getOwnPropertyDescriptor(window, 'declared')).configurable // false.
But all the variables are declared in the same class as read-only properties and all of them are assigned inside a method which is called in the constructor. Declaration of properties: protected Color m_VariableName; public Color VariableName. get { return m_VariableName; } set { } Constructor code: